I’ve been going around and around with this for hours and can’t seem to get it. I have a JavaScript function that produces a multidimentional object. I then convert it to JSON with JSON.stringify and pass it to my server connect with dmx.parse. I can’t for the life of me figure out how to access it properly in the server connect. I’ve done a simplified version below of the function.
Is this the right way to pass the JSON? And how do I access this on the server side? I’ve tried everything I can think of to no avail.
The stringified json looks like this…
[{“temp_1”:1,“temp_2”:2},{“temp_1”:2,“temp_2”:3},{“temp_1”:3,“temp_2”:4},{“temp_1”:4,“temp_2”:5},{“temp_1”:5,“temp_2”:6},{“temp_1”:6,“temp_2”:7},{“temp_1”:7,“temp_2”:8},{“temp_1”:8,“temp_2”:9},{“temp_1”:9,“temp_2”:10},{“temp_1”:10,“temp_2”:11}]
Thanks in advance for any help!
function create_array(){
var my_array = [];
var x;
var y;
for(let i = 0; i < 10; i++){
my_array[i] = {
temp_1: i + 1,
temp_2: i + 2
}
}
var my_new_array = JSON.stringify(my_array);
dmx.parse("serverconnect1.load({my_array:'" + my_new_array + "'})");
}
Step 1.
Create a variable in your server connect and use set value and set outputs to true.
Then share what’s actually being received by the SC.
Ok, so here’s a summary of the key concepts is (pseudo)code:
// Return a JS array events that have been selected
function getNewlySelectedDates() {
return yearcal.getDataSource()
.filter(item => item.workday_profile_id === '-1')
.map(item => item.startDate.getFullYear() + "-" + ("0"+(item.startDate.getMonth()+1)).slice(-2) + "-" + ("0" + item.startDate.getDate()).slice(-2));
}
function saveUserWorkdayProfile() {
let new_events = getNewlySelectedDates();
dmx.parse("var_selected_dates.setValue("+ new_events.length + ")");
dmx.parse("var_selected_date_list.setValue('" + new_events + "')");
dmx.parse("sc_check_workday_appointments.unselected_dates.setValue('" + cleared_events + "')");
}
/* - On the SC end --/
Setvalue _dates = {{ $_POST.selected_dates.split(’,’).toJSON() }}
Thanks for helping!
is .toJSON() a custom formatter? I don’t have that in my formatters list in server connect.
It’s there. Undocumented sadly.
That throws a generic 500 error with no further information…
What’s the value of string_arr when output?
And in PHP I think its php_encode rather than tojson()
str_array doesn’t output. trying to use .toJSON() there causes it to throw the error. If I just do the straight $_GET[‘my_array’] it outputs the same as the input…
[{“temp_1”:1,“temp_2”:2},{“temp_1”:2,“temp_2”:3},{“temp_1”:3,“temp_2”:4},{“temp_1”:4,“temp_2”:5},{“temp_1”:5,“temp_2”:6},{“temp_1”:6,“temp_2”:7},{“temp_1”:7,“temp_2”:8},{“temp_1”:8,“temp_2”:9},{“temp_1”:9,“temp_2”:10},{“temp_1”:10,“temp_2”:11}]
I’'m using javascript on the front end.
AHA!!! I think I got it!!
{{$_GET.my_array.parseJSON()}}
Thanks so much for your help @scalaris !
Just in case anyone is looking at this for a solution in the future. Here’s how you use can use a javascript array (object) in a server connect.
-
Create an API action that receives your array as a $_GET text variable.
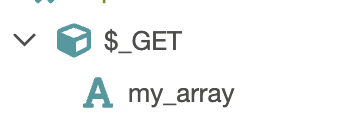
-
Create a Set Value which uses the undocumented .parseJSON to turn your string back into an array. Make sure Output is ticked
-
Click Define Schema and add your array fields here.
-
Create a Repeat Step and select your new array variable as the expression and then select your output fields (as at time of writing there was a bug where these weren’t showing up and I had to enter them manually in code view but I’d imagine that will be fixed shortly)
-
You can now use the values from the repeat step as normal. In my case I was adding the records to the database.
-
In your front end page create a Server Connect selecting your newly created API action.
-
Create your object in a javascript function. Note that this needs to be an object array, not a simple array.
function create_array(){
var my_array = [];
var x;
var y;
for(let i = 0; i < 10; i++){
my_array[i] = { //using the curly brackets here denotes it as an object
temp_1: i + 1,
temp_2: i + 2
}
}
var my_new_array = JSON.stringify(my_array); //stringify the array so it can be sent through the server action
dmx.parse("serverconnect1.load({my_array:'" + my_new_array + "'})"); //load the server connect
}
-
Run your Javascript from a flow on a button.
Voila!
3 Likes